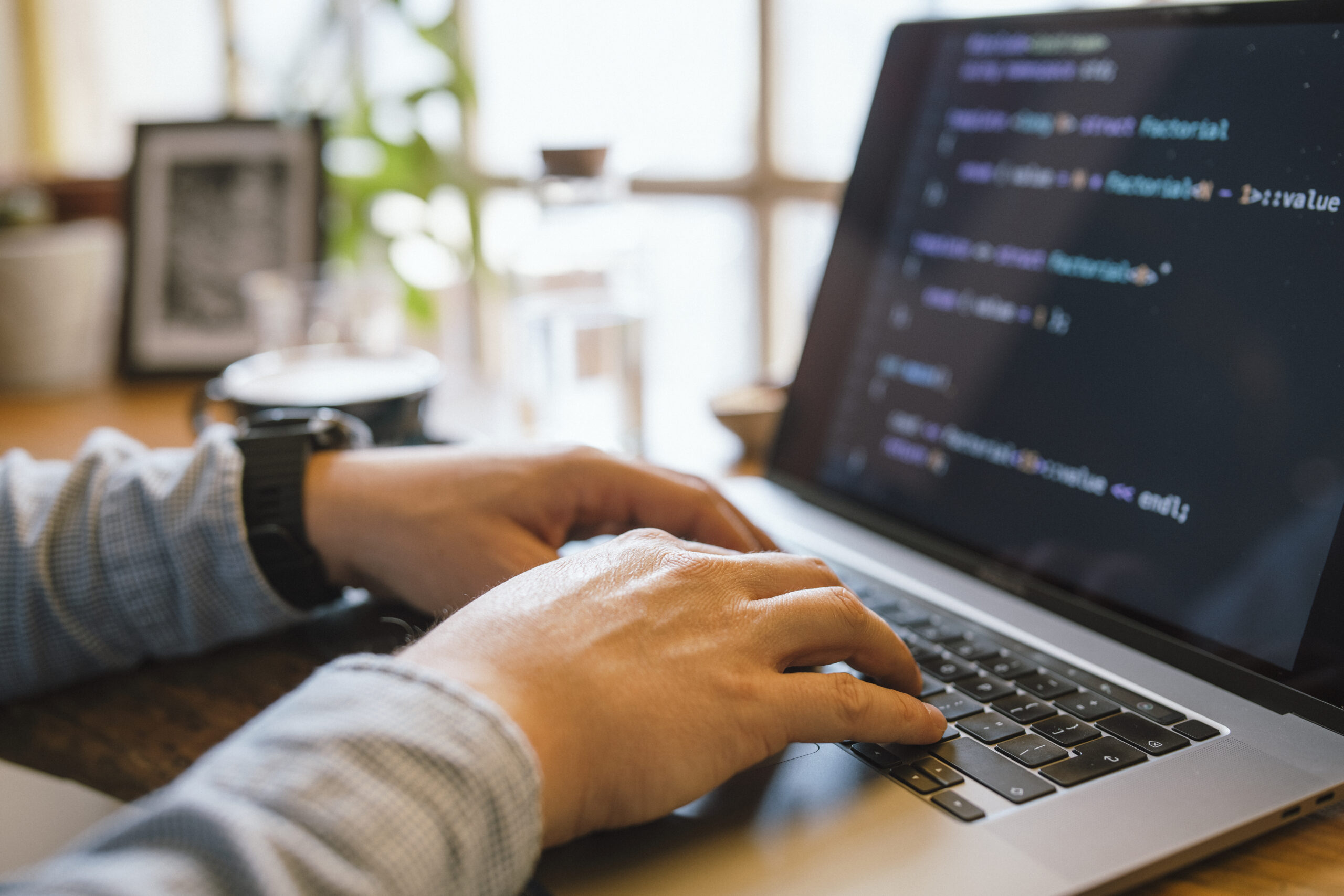
Debugging is Just about the most vital — however typically forgotten — skills inside a developer’s toolkit. It isn't pretty much correcting damaged code; it’s about understanding how and why issues go Improper, and Finding out to think methodically to solve problems efficiently. Regardless of whether you're a newbie or possibly a seasoned developer, sharpening your debugging techniques can help save hrs of annoyance and considerably transform your productivity. Here are a number of strategies to help builders stage up their debugging activity by me, Gustavo Woltmann.
Learn Your Instruments
One of several quickest ways builders can elevate their debugging techniques is by mastering the applications they use on a daily basis. Even though composing code is 1 part of enhancement, knowing ways to communicate with it efficiently during execution is Similarly critical. Present day growth environments arrive equipped with impressive debugging capabilities — but numerous builders only scratch the area of what these equipment can do.
Acquire, as an example, an Built-in Growth Natural environment (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications let you established breakpoints, inspect the value of variables at runtime, move as a result of code line by line, and in some cases modify code around the fly. When applied appropriately, they Permit you to observe particularly how your code behaves through execution, that's a must have for tracking down elusive bugs.
Browser developer instruments, like Chrome DevTools, are indispensable for entrance-stop builders. They permit you to inspect the DOM, watch network requests, check out serious-time functionality metrics, and debug JavaScript inside the browser. Mastering the console, resources, and community tabs can change discouraging UI problems into manageable tasks.
For backend or technique-level developers, applications like GDB (GNU Debugger), Valgrind, or LLDB supply deep Regulate around running processes and memory administration. Learning these resources could possibly have a steeper learning curve but pays off when debugging performance problems, memory leaks, or segmentation faults.
Outside of your IDE or debugger, come to be comfy with Edition Management systems like Git to be aware of code record, discover the exact second bugs ended up released, and isolate problematic changes.
In the long run, mastering your applications means going beyond default settings and shortcuts — it’s about establishing an intimate understanding of your growth natural environment so that when issues arise, you’re not lost at nighttime. The higher you already know your instruments, the greater time you may expend resolving the actual problem rather than fumbling through the process.
Reproduce the Problem
One of the most critical — and sometimes disregarded — measures in successful debugging is reproducing the trouble. Ahead of jumping to the code or earning guesses, builders want to create a constant environment or state of affairs wherever the bug reliably seems. With no reproducibility, fixing a bug becomes a activity of possibility, usually leading to wasted time and fragile code changes.
The initial step in reproducing a difficulty is gathering just as much context as you can. Ask questions like: What steps resulted in The difficulty? Which setting was it in — improvement, staging, or output? Are there any logs, screenshots, or mistake messages? The greater detail you may have, the less complicated it gets to be to isolate the precise situations less than which the bug takes place.
As soon as you’ve collected plenty of info, seek to recreate the trouble in your neighborhood natural environment. This could necessarily mean inputting the identical details, simulating related user interactions, or mimicking technique states. If the issue seems intermittently, contemplate crafting automated exams that replicate the sting instances or point out transitions included. These checks not just enable expose the issue but in addition reduce regressions Later on.
Sometimes, The problem can be environment-certain — it'd happen only on specific running techniques, browsers, or underneath individual configurations. Utilizing equipment like Digital equipment, containerization (e.g., Docker), or cross-browser tests platforms could be instrumental in replicating these bugs.
Reproducing the condition isn’t just a stage — it’s a frame of mind. It requires persistence, observation, and also a methodical strategy. But when you finally can continuously recreate the bug, you're currently halfway to fixing it. Using a reproducible scenario, You should use your debugging resources a lot more proficiently, exam potential fixes safely, and communicate extra clearly with your team or customers. It turns an abstract complaint right into a concrete challenge — and that’s exactly where developers prosper.
Browse and Have an understanding of the Mistake Messages
Mistake messages tend to be the most respected clues a developer has when something goes wrong. Rather then looking at them as discouraging interruptions, developers must understand to deal with error messages as immediate communications with the technique. They usually tell you what precisely happened, where by it took place, and at times even why it happened — if you know the way to interpret them.
Start off by reading through the information carefully As well as in entire. Numerous builders, particularly when under time force, look at the 1st line and quickly begin making assumptions. But further while in the error stack or logs may well lie the correct root result in. Don’t just copy and paste mistake messages into serps — study and have an understanding of them 1st.
Break the error down into elements. Can it be a syntax error, a runtime exception, or simply a logic error? Will it point to a certain file and line number? What module or functionality induced it? These thoughts can guidebook your investigation and issue you toward the liable code.
It’s also beneficial to understand the terminology in the programming language or framework you’re making use of. Mistake messages in languages like Python, JavaScript, or Java often stick to predictable styles, and Studying to recognize these can considerably quicken your debugging process.
Some problems are imprecise or generic, As well as in These situations, it’s very important to examine the context during which the mistake occurred. Examine linked log entries, enter values, and recent modifications while in the codebase.
Don’t forget compiler or linter warnings possibly. These typically precede larger sized issues and provide hints about prospective bugs.
In the long run, mistake messages are usually not your enemies—they’re your guides. Understanding to interpret them the right way turns chaos into clarity, assisting you pinpoint concerns more quickly, lessen debugging time, and turn into a additional economical and self-assured developer.
Use Logging Wisely
Logging is The most highly effective instruments inside of a developer’s debugging toolkit. When made use of effectively, it offers real-time insights into how an software behaves, helping you realize what’s taking place beneath the hood with no need to pause execution or stage with the code line by line.
A great logging technique starts with understanding what to log and at what degree. Frequent logging ranges consist of DEBUG, INFO, Alert, Mistake, and Deadly. Use DEBUG for thorough diagnostic data all through progress, Details for standard activities (like effective start-ups), Alert for likely concerns that don’t split the application, Mistake for genuine difficulties, and FATAL in the event the technique can’t proceed.
Steer clear of flooding your logs with excessive or irrelevant details. An excessive amount logging can obscure critical messages and slow down your process. Target important situations, condition alterations, input/output values, and significant selection details as part of your code.
Format your log messages clearly and continuously. Incorporate context, for example timestamps, request IDs, and performance names, so it’s easier to trace difficulties in distributed devices or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs Permit you to observe how variables evolve, what circumstances are fulfilled, and what branches of logic are executed—all with out halting This system. They’re Particularly precious in production environments wherever stepping via code isn’t possible.
Moreover, use logging frameworks and applications (like Log4j, Winston, or Python’s logging module) that help log rotation, filtering, and integration with checking dashboards.
Ultimately, smart logging is about equilibrium and clarity. Having a properly-thought-out logging tactic, you are able to decrease the time it's going to take to spot concerns, get further visibility into your programs, and Enhance the overall maintainability and dependability of your respective code.
Imagine Like a Detective
Debugging is not only a complex endeavor—it's a type of investigation. To properly determine and resolve bugs, builders ought to solution the process like a detective solving a mystery. This frame of mind can help break down complicated concerns into manageable areas and observe clues logically to uncover the foundation cause.
Begin by gathering evidence. Consider the indicators of the situation: mistake messages, incorrect output, or general performance challenges. Just like a detective surveys against the law scene, collect as much related data as you may devoid of leaping to conclusions. Use logs, examination situations, and consumer reviews to piece with each other a clear picture of what’s going on.
Subsequent, type hypotheses. Inquire yourself: What could be causing this actions? Have any improvements not long ago been manufactured for the codebase? Has this challenge transpired just before under identical situation? The purpose is always to narrow down possibilities and establish likely culprits.
Then, take a look at your theories systematically. Make an effort to recreate the issue inside of a managed atmosphere. If you suspect a selected operate or ingredient, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, ask your code inquiries and let the final results lead you nearer to the truth.
Pay back near awareness to little facts. Bugs frequently disguise inside the minimum expected destinations—similar to a missing semicolon, an off-by-a single error, or maybe a race problem. Be complete and individual, resisting the urge to patch The difficulty without having fully comprehension it. Temporary fixes may possibly hide the true trouble, only for it to resurface afterwards.
Lastly, hold notes on what you experimented with and learned. Just as detectives log their investigations, documenting your debugging course of action can save time for foreseeable future issues and aid Many others realize your reasoning.
By imagining similar to a detective, developers can sharpen their analytical capabilities, solution issues methodically, and turn into more practical at uncovering concealed issues in sophisticated devices.
Write Tests
Composing assessments is among the most effective approaches to increase your debugging capabilities and In general development efficiency. Exams not merely support capture bugs early and also function a security Web that offers you self confidence when building improvements towards your codebase. A well-tested application is easier to debug because it enables you to pinpoint precisely in which and when a difficulty takes place.
Get started with device assessments, which target specific features or modules. These modest, isolated exams can swiftly reveal regardless of whether a particular bit of logic is Doing work as anticipated. Whenever a check fails, you instantly know where to glimpse, noticeably reducing some time expended debugging. Unit assessments are Specifically beneficial for catching regression bugs—problems that reappear after Beforehand staying mounted.
Subsequent, combine integration assessments and conclude-to-finish tests into your workflow. These assist ensure that several areas of your application do the job jointly easily. They’re particularly handy for catching bugs that take place in complex devices with a number of parts or solutions interacting. If a thing breaks, your exams can show you which Section of the pipeline failed and underneath what situations.
Crafting exams also forces you to definitely Feel critically regarding your code. To check a function thoroughly, you may need to know its inputs, predicted outputs, and edge instances. This standard of comprehending The natural way qualified prospects to raised code construction and less bugs.
When debugging a problem, producing a failing test that reproduces the bug might be a robust first step. When the test fails constantly, you could give attention to repairing the bug and check out your check move when the issue is settled. This tactic ensures that the identical bug doesn’t return Down the road.
In short, creating assessments turns debugging from the frustrating guessing sport into a structured and predictable course of action—aiding you capture extra bugs, faster and even more reliably.
Just take Breaks
When debugging a tough difficulty, it’s easy to become immersed in the trouble—watching your display screen for several hours, seeking solution immediately after Alternative. But one of the most underrated debugging tools is simply stepping away. Taking breaks assists you reset your thoughts, lessen annoyance, and infrequently see The difficulty from the new point of view.
When you're too near the code for too long, cognitive exhaustion sets in. You might start overlooking obvious faults or misreading code that you wrote just several hours before. With this condition, your brain will become a lot less productive at difficulty-solving. A short wander, a espresso split, or perhaps switching to a different task for ten–quarter-hour can refresh your target. Numerous developers report discovering the foundation of a challenge once they've taken time for you to disconnect, letting their subconscious do the job from the track record.
Breaks also assist prevent burnout, Primarily for the duration of for a longer time debugging sessions. Sitting down before a display, mentally trapped, is not simply unproductive but additionally draining. Stepping absent means that you can return with renewed Vitality and a clearer mentality. You would possibly abruptly notice a lacking semicolon, a logic flaw, or perhaps a misplaced more info variable that eluded you right before.
If you’re caught, a good guideline would be to established a timer—debug actively for 45–sixty minutes, then have a 5–ten minute split. Use that time to move around, stretch, or do a little something unrelated to code. It may well truly feel counterintuitive, Primarily beneath limited deadlines, nevertheless it basically results in speedier and more effective debugging In the long term.
In short, getting breaks is not a sign of weak point—it’s a sensible method. It gives your brain Place to breathe, improves your viewpoint, and can help you avoid the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and relaxation is an element of solving it.
Find out From Just about every Bug
Each bug you come upon is more than just A brief setback—It can be a possibility to develop like a developer. Irrespective of whether it’s a syntax error, a logic flaw, or even a deep architectural situation, every one can instruct you some thing useful in case you go to the trouble to mirror and examine what went Erroneous.
Get started by inquiring yourself a couple of crucial inquiries when the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with improved practices like device tests, code reviews, or logging? The answers usually reveal blind spots inside your workflow or knowing and enable you to Construct more powerful coding behavior shifting forward.
Documenting bugs can also be an excellent habit. Keep a developer journal or manage a log in which you Observe down bugs you’ve encountered, the way you solved them, and That which you uncovered. After a while, you’ll start to see patterns—recurring issues or common issues—you can proactively keep away from.
In crew environments, sharing Everything you've learned from the bug with the peers can be Primarily highly effective. No matter if it’s by way of a Slack concept, a short produce-up, or a quick knowledge-sharing session, encouraging Other folks avoid the exact situation boosts group performance and cultivates a stronger Mastering tradition.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as crucial aspects of your growth journey. In the end, many of the greatest builders usually are not those who create fantastic code, but people who consistently find out from their issues.
Ultimately, Just about every bug you repair provides a new layer to the talent set. So future time you squash a bug, take a minute to replicate—you’ll arrive absent a smarter, extra capable developer on account of it.
Summary
Enhancing your debugging techniques takes time, follow, and endurance — but the payoff is big. It would make you a far more efficient, assured, and able developer. Another time you are knee-deep in a very mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become greater at That which you do.